本文属于机器翻译版本。若本译文内容与英语原文存在差异,则一律以英文原文为准。
使用 Amazon Cognito 用户池进行身份验证
Amazon Cognito 包含多种对用户进行身份验证的方法。用户可以使用 WebAuthn 密码和密钥登录。Amazon Cognito 可以通过电子邮件或短信向他们发送一次性密码。您可以实现 Lambda 函数来编排您自己的挑战和响应顺序。这些是身份验证流程。在身份验证流程中,用户提供密钥,然后 Amazon Cognito 会验证该密钥,然后发放 JSON 网络令牌 (JWTs) 供应用程序使用 OIDC 库进行处理。在本章中,我们将讨论如何为各种应用程序环境中的各种身份验证流程配置用户池和应用程序客户端。您将了解使用托管登录的托管登录页面的选项,以及在 Amazon SDK 中构建自己的逻辑和前端的选项。
所有用户群体(无论您是否具有域)都可以在用户群体 API 中对用户进行身份验证。如果您向用户群体添加域,则可以使用用户群体端点。用户群体 API 支持针对 API 请求的各种授权模型和请求流程。
为了验证用户的身份,Amazon Cognito 支持除了电子邮件和短信、一次性密码和密钥等密码之外还包含质询类型的身份验证流程。
实现身份验证流程
无论您是在实现托管登录,还是使用用于身份验证的 S Amazon DK 的自定义应用程序前端,都必须针对要实现的身份验证类型配置应用程序客户端。以下信息描述了应用程序客户端和应用程序中身份验证流程的设置。
关于使用用户池进行身份验证的注意事项
在使用 Amazon Cognito 用户池设计身份验证模型时,请考虑以下信息。
- 托管登录和托管 UI 中的身份验证流程
-
托管登录和经典托管用户界面有不同的身份验证选项。在托管登录中,您只能进行无密码和密钥身份验证。
- 自定义身份验证流程仅在 Amazon SDK 身份验证中可用
-
您无法使用托管登录或经典托管 UI 进行自定义身份验证流程,也无法使用 Lambda 触发器进行自定义身份验证。自定义身份验证可在使用进行身份验证时使用 Amazon SDKs。
- 外部身份提供商 (IdP) 登录的托管登录
-
在使用身份验证时,您无法通过第三方 IdPs登录用户 Amazon SDKs。您必须实现托管登录或经典托管用户界面,重定向到 IdPs,然后在应用程序中使用 OIDC 库处理生成的身份验证对象。有关托管登录的更多信息,请参阅用户池托管登录。
- 无密码身份验证对其他用户功能的影响
-
使用用户池和应用程序客户端中的一次性密码或密钥激活无密码登录会影响用户的创建和迁移。启用无密码登录时:
-
管理员可以创建没有密码的用户。默认的邀请消息模板更改为不再包含
{###}
密码占位符。有关更多信息,请参阅 以管理员身份创建用户账户。 -
对于基于 SDK 的SignUp操作,用户在注册时无需提供密码。即使允许使用无密码身份验证,托管登录和托管用户界面也需要在注册页面中输入密码。有关更多信息,请参阅 注册并确认用户账户。
-
从 CSV 文件导入的用户可以立即使用无密码选项登录,而无需重置密码,前提是他们的属性包括可用无密码登录选项的电子邮件地址或电话号码。有关更多信息,请参阅 通过 CSV 文件将用户导入用户池中。
-
无密码身份验证不会调用用户迁移 Lambda 触发器。
-
使用无密码第一因素登录的用户无法在会话中添加多重身份验证 (MFA) 因素。只有基于密码的身份验证流程支持 MFA。
-
- Passkey 依赖方 URLs 不能出现在公共后缀列表中
-
在密钥配置中,您可以使用自己拥有的域名作为信赖方 (RP) ID。
www.example.com
此配置旨在支持在您拥有的域上运行的自定义应用程序。公共后缀列表(PSL) 包含受保护的高级域。当你尝试将 RP 网址设置为 PSL 上的域名时,Amazon Cognito 会返回错误。
身份验证会话流持续时间
根据用户池的功能,您最终可能会在应用程序从 Amazon Cognito 检索令牌RespondToAuthChallenge
之前或之前对InitiateAuth
多个挑战做出响应。Amazon Cognito 在对每个请求的响应中都包含一个会话字符串。要将您的 API 请求合并到身份验证流程中,请在每个后续请求中包含来自上一个请求的响应中的会话字符串。默认情况下,在会话字符串过期之前,您的用户有三分钟时间完成每项质询。要调整此时段,请更改您的应用程序客户端的 Authentication flow session duration(身份验证流程会话持续时间)。以下过程介绍如何在应用程序客户端配置中更改此设置。
注意
身份验证流程会话持续时间设置适用于使用 Amazon Cognito 用户群体 API 进行身份验证。托管登录将多因素身份验证的会话持续时间设置为 3 分钟,密码重置代码的会话持续时间设置为 8 分钟。
有关应用程序客户端的更多信息,请参阅特定于应用程序的应用程序客户端设置。
登录尝试失败时的锁定行为
在使用密码进行五次未经身份验证或 IAM 授权的登录尝试失败后,Amazon Cognito 会将您的用户锁定一秒钟。然后,每多一次失败的尝试,锁定持续时间将增加一倍,最长约为 15 分钟。在锁定期内进行的尝试会产生 Password attempts exceeded
异常,不会影响后续锁定期的持续时间。对于累计 n 次的失败登录尝试(不包括 Password attempts
exceeded
异常),Amazon Cognito 会将您的用户锁定 2^(n-5) 秒。要将锁定重置为其 n=0 初始状态,用户必须在锁定期到期后才能成功登录,或者在锁定后连续 15 分钟的任何时间内都不得发起任何登录尝试。此行为随时可能会发生变化。此行为不适用于自定义质询,除非它们还执行基于密码的身份验证。
身份验证会话示例
下图和 step-by-step指南说明了用户登录应用程序的典型场景。示例应用程序为用户提供了多个登录选项。他们通过输入凭据来选择一个,提供额外的身份验证因素,然后登录。
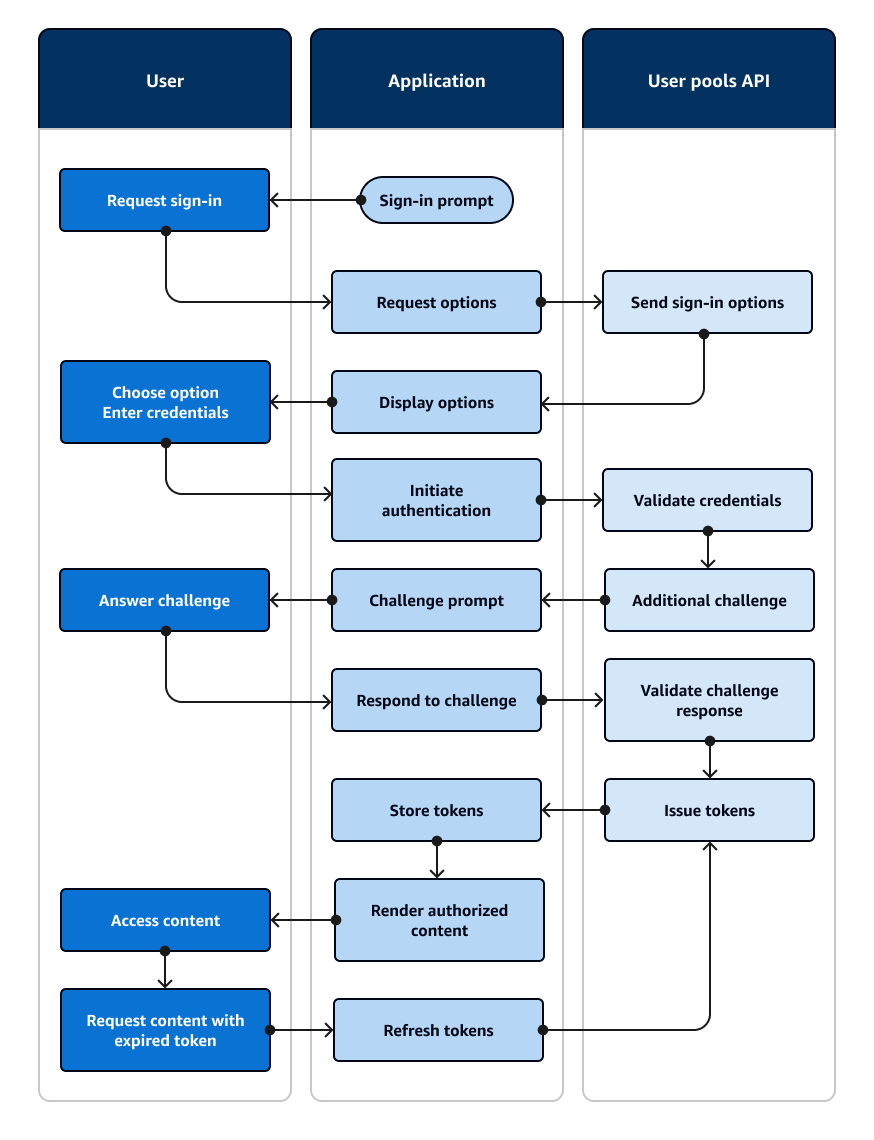
想象一下带有登录页面的应用程序,用户可以在其中使用用户名和密码登录、在电子邮件中请求一次性验证码或选择指纹选项。
-
登录提示:您的应用程序显示带有 “登录” 按钮的主屏幕。
-
请求登录:用户选择 “登录”。您的应用程序会从 Cookie 或缓存中检索他们的用户名,或者提示他们输入用户名。
-
请求选项:您的应用通过
USER_AUTH
流程中的InitiateAuth
API 请求来请求用户的登录选项,请求用户可用的登录方法。 -
发送登录选项:Amazon Cognito 回复
PASSWORD
为EMAIL_OTP
、和。WEB_AUTHN
该响应包含一个会话标识符,供您在下一个响应中回放。 -
显示选项:您的应用程序显示用户界面元素,供用户输入用户名和密码、获取一次性代码或扫描指纹。
-
选择选项/输入凭证:用户输入其用户名和密码。
-
启动身份验证:您的应用程序通过一个
RespondToAuthChallenge
API 请求向用户的登录信息提供确认用户名密码登录并提供用户名和密码。 -
验证凭证:Amazon Cognito 确认用户的证书。
-
其他挑战:用户使用身份验证器应用程序配置了多因素身份验证。亚马逊 Cognito 回来了一项挑战。
SOFTWARE_TOKEN_MFA
-
质询提示:您的应用程序会显示一个表单,要求用户的身份验证器应用程序提供基于时间的一次性密码 (TOTP)。
-
回答挑战:用户提交 TOTP。
-
回应质疑:在另一个
RespondToAuthChallenge
请求中,您的应用程序会提供用户的 TOTP。 -
验证质询响应:Amazon Cognito 确认用户的代码,并确定您的用户池已配置为不向当前用户发出其他质询。
-
发行令牌:Amazon Cognito 会返回 ID、访问权限和刷新 JSON 网络令牌 () JWTs。用户的初始身份验证已完成。
-
存储令牌:您的应用程序会缓存用户的令牌,以便它可以引用用户数据,授权访问资源,并在令牌到期时更新令牌。
-
呈现授权内容:您的应用程序根据用户的身份和角色确定其对资源的访问权限,并提供应用程序内容。
-
访问内容:用户已登录并开始使用该应用程序。
-
使用过期令牌请求内容:稍后,用户请求需要授权的资源。用户的缓存令牌已过期。
-
刷新令牌:您的应用程序使用用户保存的刷新令牌
InitiateAuth
发出请求。 -
发行令牌:亚马逊 Cognito 会返回新的 ID 和访问权限。 JWTs用户会话可以安全刷新,无需额外提示输入凭据。
您可以使用Amazon Lambda 触发器来自定义用户身份验证的方式。作为身份验证流程的一部分,这些触发器将发布并验证自己的质询。
您还可以在安全后端服务器上对用户使用管理员身份验证流程。您可以使用用户迁移身份验证流程来实现用户迁移,而无需用户重置密码。