Authenticating requests for Amazon SQS
Authentication is the process of identifying and verifying the party that sends a request.
During the first stage of authentication, Amazon verifies the identity of the producer and
whether the producer is registered to use Amazon
-
The producer (sender) obtains the necessary credential.
-
The producer sends a request and the credential to the consumer (receiver).
-
The consumer uses the credential to verify whether the producer sent the request.
-
One of the following happens:
-
If authentication succeeds, the consumer processes the request.
-
If authentication fails, the consumer rejects the request and returns an error.
-
Basic authentication process with HMAC-SHA
When you access Amazon SQS using the Query API, you must provide the following items to authenticate your request:
-
The Amazon Access Key ID that identifies your Amazon Web Services account, which Amazon uses to look up your Secret Access Key.
-
The HMAC-SHA request signature, calculated using your Secret Access Key (a shared secret known only to you and Amazon—for more information, see RFC2104
). The Amazon SDK handles the signing process; however, if you submit a query request over HTTP or HTTPS, you must include a signature in every query request. -
Derive a Signature Version 4 Signing Key. For more information, see Deriving the Signing Key with Java.
Note
Amazon SQS supports Signature Version 4, which provides improved SHA256-based security and performance over previous versions. When you create new applications that use Amazon SQS, use Signature Version 4.
-
Base64-encode the request signature. The following sample Java code does this:
package amazon.webservices.common; // Define common routines for encoding data in Amazon requests. public class Encoding { /* Perform base64 encoding of input bytes. * rawData is the array of bytes to be encoded. * return is the base64-encoded string representation of rawData. */ public static String EncodeBase64(byte[] rawData) { return Base64.encodeBytes(rawData); } }
-
-
The timestamp (or expiration) of the request. The timestamp that you use in the request must be a
dateTime
object, with the complete date, including hours, minutes, and seconds. For example: 2007-01-31T23:59:59Z
Although this isn't required, we recommend providing the object using the Coordinated Universal Time (Greenwich Mean Time) time zone.Note
Make sure that your server time is set correctly. If you specify a timestamp (rather than an expiration), the request automatically expires 15 minutes after the specified time (Amazon doesn't process requests with timestamps more than 15 minutes earlier than the current time on Amazon servers).
If you use .NET, you must not send overly specific timestamps (because of different interpretations of how extra time precision should be dropped). In this case, you should manually construct
dateTime
objects with precision of no more than one millisecond.
Part 1: The request from the user
The following is the process you must follow to authenticate Amazon requests using an HMAC-SHA request signature.
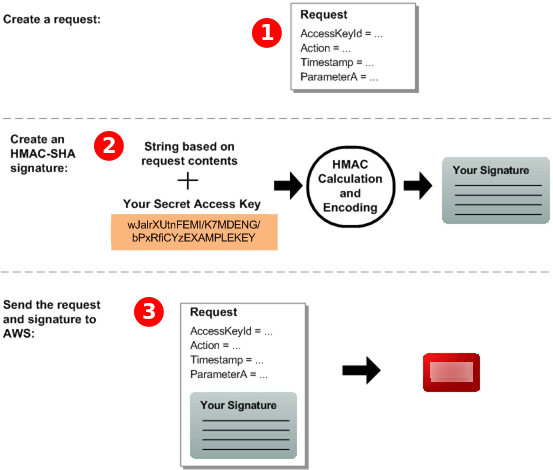
-
Construct a request to Amazon.
-
Calculate a keyed-hash message authentication code (HMAC-SHA) signature using your Secret Access Key.
-
Include the signature and your Access Key ID in the request, and then send the request to Amazon.
Part 2: The response from Amazon
Amazon begins the following process in response.
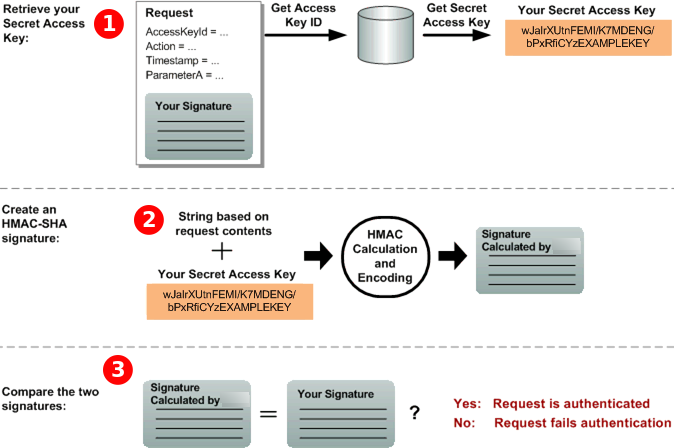
-
Amazon uses the Access Key ID to look up your Secret Access Key.
-
Amazon generates a signature from the request data and the Secret Access Key, using the same algorithm that you used to calculate the signature you sent in the request.
-
One of the following happens:
-
If the signature that Amazon generates matches the one you send in the request, Amazon considers the request to be authentic.
-
If the comparison fails, the request is discarded, and Amazon returns an error.
-