Learn how to use Amazon Cloud Map service discovery with custom attributes
This tutorial demonstrates how you can use Amazon Cloud Map service discovery with custom attributes that are discoverable using the Amazon Cloud Map API. This tutorial walks you through creating a client application in an Amazon Cloud9 environment that uses two Lambda functions to write data to a DynamoDB table and then read from the table. The Lambda functions and DynamoDB table are registered in Amazon Cloud Map as service instances. The code in the client application and Lambda functions uses Amazon Cloud Map custom attributes to discover the resources needed to perform the job.
The following diagram demonstrates the high level architecture this tutorial uses.
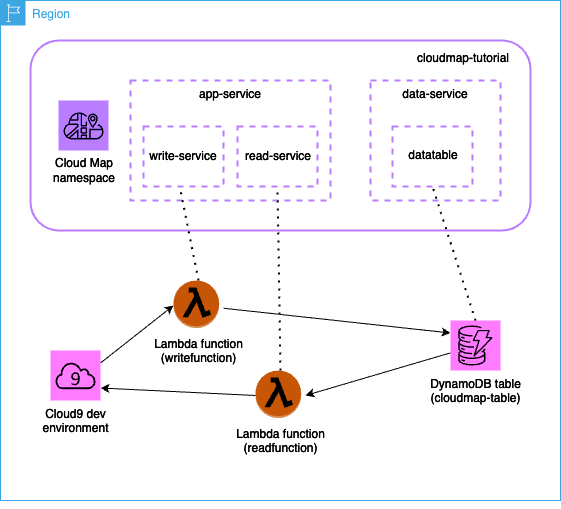
Important
You will create Amazon resources during the workshop which will incur a cost in your Amazon account. It is recommended to clean-up the resources as soon as you finish the workshop to minimize the cost.
Prerequisites
Before you begin, complete the steps in Set up to use Amazon Cloud Map.
Step 1: Create an Amazon Cloud Map namespace
In this step, you create an Amazon Cloud Map namespace. A namespace is a construct used to group services for an application. When you create the namespace, you specify how the resources will be discoverable. For this tutorial, the resources created in this namespace will be discoverable with Amazon Cloud Map API calls using custom attributes. You will learn about this more in a later step.
Sign in to the Amazon Web Services Management Console and open the Amazon Cloud Map console at https://console.amazonaws.cn/cloudmap/
. -
Choose Create namespace.
-
For Namespace name, specify
cloudmap-tutorial
. -
(Optional) For Namespace description, specify a description for what you intend to use the namespace for.
-
For Instance discovery, select API calls.
-
Leave the rest of the default values and choose Create namespace.
Step 2: Create a DynamoDB table
In this step, you create a DynamoDB table which is used to store and retrieve data for the sample application created later in this tutorial.
For information about how to create an DynamoDB, see Step 1: Create a table in the DynamoDB Developer Guide and use the following table to determine what options to specify.
Option | Value |
---|---|
Table name |
cloudmap |
Partition key |
id |
Keep the default values for the rest of the settings and create the table.
Step 3: Create the Amazon Cloud Map data service
In this step, you create a Amazon Cloud Map service and then register the DynamoDB table created in the last step as a service instance.
-
Open the Amazon Cloud Map console at https://console.amazonaws.cn/cloudmap/
-
From the list of namespaces, select the
cloudmap-tutorial
namespace and choose View details. -
In the Services section, choose Create service and do the following.
-
For Service name, enter
data-service
. -
Leave the rest of the default values and choose Create service.
-
-
In the Services section, select the
data-service
service and choose View details. -
In the Service instances section, choose Register service instance.
-
On the Register service instance page, do the following.
-
For Instance type, select Identifying information for another resource.
-
For Service instance id, specify
data-instance
. -
In the Custom attributes section, specify the following key-value pairs.
-
key =
name
, value =datatable
-
key =
tablename
, value =cloudmap
-
-
Verify the attributes match the image below and choose Register service instance.
-
Step 4: Create an Amazon Lambda execution role
In this step, you create an IAM role that the Amazon Lambda function we create in the next
step uses. You can name the role cloudmap-role
and omit the permissions boundary as
this IAM role is only used for this tutorial and you can delete it afterwards.
To create the service role for Lambda (IAM console)
Sign in to the Amazon Web Services Management Console and open the IAM console at https://console.amazonaws.cn/iam/
. -
In the navigation pane of the IAM console, choose Roles, and then choose Create role.
-
For Trusted entity type, choose Amazon Web Service.
-
For Service or use case, choose Lambda, and then choose the Lambda use case.
-
Choose Next.
-
Search for, and select the box next to, the
PowerUserAccess
policy and then choose Next. -
Choose Next.
-
For Role name, specify
cloudmap-tutorial-role
. -
Review the role, and then choose Create role.
Step 5: Create the Lambda function to write data
In this step, you create a Lambda function authored from scratch that writes data to the DynamoDB table by using the Amazon Cloud Map API to query the Amazon Cloud Map service you created.
For information about creating a Lambda function, see Create a Lambda function with the console in the Amazon Lambda Developer Guide and use the following table to determine what options to specify or choose.
Option | Value |
---|---|
Function name |
writefunction |
Runtime |
Python 3.12 |
Architecture |
x86_64 |
Permissions |
Use an existing role |
Existing role |
cloudmap-tutorial-role |
After you create the function, update the example code to reflect the following Python code,
and then deploy the function. Note that you're specifying the datatable
custom
attribute you associated with the Amazon Cloud Map service instance you created for the DynamoDB table.
import json import boto3 import random def lambda_handler(event, context): serviceclient = boto3.client('servicediscovery') response = serviceclient.discover_instances( NamespaceName='cloudmap-tutorial', ServiceName='data-service', QueryParameters={ 'name': 'datatable' }) tablename = response["Instances"][0]["Attributes"]["tablename"] dynamodbclient = boto3.resource('dynamodb') table = dynamodbclient.Table(tablename) response = table.put_item( Item={ 'id': str(random.randint(1,100)), 'todo': event }) return { 'statusCode': 200, 'body': json.dumps(response) }
Step 6: Create the Amazon Cloud Map app service
In this step, you create an Amazon Cloud Map service and then register the Lambda write function as a service instance.
-
Open the Amazon Cloud Map console at https://console.amazonaws.cn/cloudmap/
-
In the left navigation, choose Namespaces.
-
From the list of namespaces, select the
cloudmap-tutorial
namespace and choose View details. -
In the Services section, choose Create service and do the following.
-
For Service name, enter
app-service
. -
Leave the rest of the default values and choose Create service.
-
-
In the Services section, select the
app-service
service and choose View details. -
In the Service instances section, choose Register service instance.
-
On the Register service instance page, do the following.
-
For Instance type, select Identifying information for another resource.
-
For Service instance id, specify
write-instance
. -
In the Custom attributes section, specify the following key-value pairs.
-
key =
name
, value =writeservice
-
key =
function
, value =writefunction
-
-
Verify the attributes match the image below and choose Register service instance.
-
Step 7: Create the Lambda function to read data
In this step, you create a Lambda function authored from scratch that writes data to the DynamoDB table you created.
For information about creating a Lambda function, see Create a Lambda function with the console in the Amazon Lambda Developer Guide and use the following table to determine what options to specify or choose.
Option | Value |
---|---|
Function name |
readfunction |
Runtime |
Python 3.12 |
Architecture |
x86_64 |
Permissions |
Use an existing role |
Existing role |
cloudmap-tutorial-role |
After you create the function, update the example code to reflect the following Python code, and then deploy the function.
import json import boto3 def lambda_handler(event, context): serviceclient = boto3.client('servicediscovery') response = serviceclient.discover_instances(NamespaceName='cloudmap-tutorial', ServiceName='data-service', QueryParameters={ 'name': 'datatable' }) tablename = response["Instances"][0]["Attributes"]["tablename"] dynamodbclient = boto3.resource('dynamodb') table = dynamodbclient.Table(tablename) response = table.get_item(Key={'id': event}) return { 'statusCode': 200, 'body': json.dumps(response) }
Step 8: Create an Amazon Cloud Map service instance
In this step, you register the Lambda read function as a service instance in the
app-service
service you previously created.
-
Open the Amazon Cloud Map console at https://console.amazonaws.cn/cloudmap/
-
In the left navigation, choose Namespaces.
-
From the list of namespaces, select the
cloudmap-tutorial
namespace and choose View details. -
In the Services section, select the
app-service
service and choose View details. -
In the Service instances section, choose Register service instance.
-
On the Register service instance page, do the following.
-
For Instance type, select Identifying information for another resource.
-
For Service instance id, specify
read-instance
. -
In the Custom attributes section, specify the following key-value pairs.
-
key =
name
, value =readservice
-
key =
function
, value =readfunction
-
-
Verify the attributes match the image below and choose Register service instance.
-
Step 9: Create a development environment
Amazon Cloud9 is an integrated development environment (IDE) managed by Amazon. The Amazon Cloud9 IDE provides the software and toooling needed for dynamic programming. In this step, we create an Amazon Cloud9 environment and configure it with the Amazon SDK for Python (Boto3) which you will to program with the Amazon API.
For information about creating a Amazon Cloud9 environment, see Creating an EC2 environment in the Amazon Cloud9 User Guide and use the following table to determine what options to specify or choose.
Option | Value |
---|---|
Name |
cloudmap-tutorial |
Environment type |
New EC2 instance |
Instance type |
t2.micro |
Platform |
Ubuntu Server 22.04 LTS |
Leave the rest of the default selections unchanged. Create the environment and then open it in Amazon Cloud9. This provides you with a bash shell to work with.
Important
If you have issues opening your Amazon Cloud9 environment, see Amazon Cloud9 troubleshooting: Can't open an environment in the Amazon Cloud9 User Guide.
Using the bash shell, run the following commands to configure the environment.
-
Update the environment.
sudo apt-get -y update
-
Verify that
python3
is installed.python3 --version
-
Install the Boto3 package in the environment.
sudo apt install -y python3-boto3
Step 10: Create a frontend client
Using the Amazon Cloud9 development environment created in the previous step, you create a frontend client that uses code that discovers the services you configured in Amazon Cloud Map and makes calls to these services.
-
In the Amazon Cloud9 environment, in the File menu, choose New file. This creates a file named
Untitled1
. -
In the
Untitled1
file, copy and paste the following code. This code discovers the Lambda function to write data by searching for the custom attributename=writeservice
in theapp-service
service. The name of the Lambda function is returned which is responsible for writing data to the DynamoDB table. Then the Lambda function is invoked, passing a sample payload.import boto3 serviceclient = boto3.client('servicediscovery') response = serviceclient.discover_instances(NamespaceName='cloudmap-tutorial', ServiceName='app-service', QueryParameters={ 'name': 'writeservice' }) functionname = response["Instances"][0]["Attributes"]["function"] lambdaclient = boto3.client('lambda') resp = lambdaclient.invoke(FunctionName=functionname, Payload='"This is a test data"') print(resp["Payload"].read())
-
From the File menu, choose Save As... and save the file as
writeclient.py
. -
From the bash shell in your Amazon Cloud9 environment, use the following command to run the Python code.
python3 writeclient.py
The output should be a
200
response, similar to the following.b'{"statusCode": 200, "body": "{\\"ResponseMetadata\\": {\\"RequestId\\": \\"Q0M038IT0BPBVBJK8OCKK6I6M7VV4KQNSO5AEMVJF66Q9ASUAAJG\\", \\"HTTPStatusCode\\": 200, \\"HTTPHeaders\\": {\\"server\\": \\"Server\\", \\"date\\": \\"Wed, 06 Mar 2024 22:46:09 GMT\\", \\"content-type\\": \\"application/x-amz-json-1.0\\", \\"content-length\\": \\"2\\", \\"connection\\": \\"keep-alive\\", \\"x-amzn-requestid\\": \\"Q0M038IT0BPBVBJK8OCKK6I6M7VV4KQNSO5AEMVJF66Q9ASUAAJG\\", \\"x-amz-crc32\\": \\"2745614147\\"}, \\"RetryAttempts\\": 0}}"}'
Note
If the output is an error message that says the task has timed out, update the
writefunction
Lambda function's timeout value. For more information, see Configure Lambda function timeout in the Amazon Lambda Developer Guide. -
To verify the write was successful in the previous step, create a read client.
Sign in to the Amazon Web Services Management Console and open the DynamoDB console at https://console.amazonaws.cn/dynamodb/
. -
In the left navigation pane, choose Tables.
-
From the list of tables, select your cloudmap-table and use the Actions menu to choose Explore items.
-
In the Items returned section, note the numerical value in the id(String) column.
The following shows an example, where the id(String) value is
98
. -
In the Amazon Cloud9 environment, in the File menu, choose New file which creates a file named
Untitled1
. -
In the
Untitled1
file, copy and paste the following code. Replace thePayload
value with theid (String)
value from your DynamoDB table in the previous step. This code reads from the table and will return the value that you wrote to the table in the previous step.import boto3 serviceclient = boto3.client('servicediscovery') response = serviceclient.discover_instances(NamespaceName='cloudmap-tutorial', ServiceName='app-service', QueryParameters={ 'name': 'readservice' }) functionname = response["Instances"][0]["Attributes"]["function"] lambdaclient = boto3.client('lambda') resp = lambdaclient.invoke(FunctionName=functionname, InvocationType='RequestResponse', Payload='"
98
"') print(resp["Payload"].read()) -
From the File menu, choose Save As... and save the file as
readclient.py
. -
From the bash shell in your Amazon Cloud9 environment, use the following command to run the Python code.
python3 readclient.py
The output should look similar to the following.
b'{"statusCode": 200, "body": "{\\"Item\\": {\\"id\\": \\"98\\", \\"todo\\": \\"This is a test data\\"}, \\"ResponseMetadata\\": {\\"RequestId\\": \\"JSO5DLRGF0JUPQN4NCH369ABMBVV4KQNSO5AEMVJF66Q9ASUAAJG\\", \\"HTTPStatusCode\\": 200, \\"HTTPHeaders\\": {\\"server\\": \\"Server\\", \\"date\\": \\"Wed, 06 Mar 2024 23:03:38 GMT\\", \\"content-type\\": \\"application/x-amz-json-1.0\\", \\"content-length\\": \\"61\\", \\"connection\\": \\"keep-alive\\", \\"x-amzn-requestid\\": \\"JSO5DLRGF0JUPQN4NCH369ABMBVV4KQNSO5AEMVJF66Q9ASUAAJG\\", \\"x-amz-crc32\\": \\"3104232745\\"}, \\"RetryAttempts\\": 0}}"}'
Note
If the output is an error message that says the task has timed out, update the
readfunction
Lambda function's timeout value. For more information, see Configure Lambda function timeout in the Amazon Lambda Developer Guide.
Step 11: Clean up the resources
Once you have completed the tutorial, delete the resources to avoid incurring additional charges. Amazon Cloud Map requires that you clean them up in reverse order, the service instances first, then the services, and finally the namespace. The following steps walk you through cleaning up the Amazon Cloud Map resources used in this tutorial.
To delete the Amazon Cloud Map resources
Sign in to the Amazon Web Services Management Console and open the Amazon Cloud Map console at https://console.amazonaws.cn/cloudmap/
. -
From the list of namespaces, select the
cloudmap-tutorial
namespace and choose View details. -
On the namespace details page, from the list of services, select the
data-service
service and choose View details. -
In the Service instances section, select the
data-instance
instance and choose Deregister. -
Using the breadcrumb at the top of the page, select cloudmap-tutorial.com to navigate back to the namespace detail page.
-
On the namespace details page, from the list of services, select the data-service service and choose Delete.
-
Repeat steps 3-6 for the
app-service
service and thewrite-instance
andread-instance
service instances. -
In the left navigation, choose Namespaces.
-
Select the
cloudmap-tutorial
namespace and choose Delete.
The following table lists procedures that you can use to delete the other resources used in the tutorial.
Resource | Steps |
---|---|
DynamoDB table |
Step 8: (Optional) clean up resources in the Amazon DynamoDB Developer Guide |
Lambda functions and associated IAM execution role |
Clean up in the Amazon Lambda Developer Guide |
Amazon Cloud9 environment |
Deleting an environment in Amazon Cloud9 in the Amazon Cloud9 User Guide. |