本文属于机器翻译版本。若本译文内容与英语原文存在差异,则一律以英文原文为准。
包含 Spring 以及适用于 Java 的 X-Ray 开发工具包的 AOP
本主题介绍如何使用 X-Ray 开发工具包和 Spring Framework 检测应用程序,而不更改其核心逻辑。这意味着现在有一种非侵入性的方法可以检测远程运行的应用程序。 Amazon
启用 Spring 中的 AOP
配置 Spring
您可以使用 Maven 或 Gradle 将 Spring 配置为使用 AOP 检测您的应用程序。
如果您使用 Maven 来生成应用程序,则在 pom.xml
文件中添加以下依赖项。
<dependency> <groupId>com.amazonaws</groupId> <artifactId>aws-xray-recorder-sdk-spring</artifactId> <version>2.11.0</version> </dependency>
对于 Gradle,在 build.gradle
文件中添加以下依赖项。
compile 'com.amazonaws:aws-xray-recorder-sdk-spring:2.11.0'
配置 Spring Boot
除了上一节中介绍的 Spring 依赖项,如果您使用的是 Spring Boot,如果尚位在类路径上,请添加以下依赖项。
Maven:
<dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-aop</artifactId> <version>2.5.2</version> </dependency>
Gradle:
compile 'org.springframework.boot:spring-boot-starter-aop:2.5.2'
向您的应用程序添加跟踪筛选器
将 Filter
添加到 WebConfig
类。将分段名称作为字符串传递到 AWSXRayServletFilter
构造函数。有关跟踪筛选器和检测传入请求的更多信息,请参阅 使用适用于 Java 的 X-Ray 开发工具包跟踪传入请求。
例 src/main/java/myapp/WebConfig.java-春季
package myapp
;
import org.springframework.context.annotation.Configuration;
import org.springframework.context.annotation.Bean;
import javax.servlet.Filter;
import com.amazonaws.xray.javax.servlet.AWSXRayServletFilter;
@Configuration
public class WebConfig {
@Bean
public Filter TracingFilter() {
return new AWSXRayServletFilter("Scorekeep
");
}
}
Jakarta 支持
Spring 6 企业版使用 Jakarta
对于筛选器类,将 javax
替换为 jakarta
。配置分段命名策略时,如下所示,在命名策略类名称前添加 jakarta
:
package myapp; import org.springframework.context.annotation.Configuration; import org.springframework.context.annotation.Bean; import jakarta.servlet.Filter; import com.amazonaws.xray.jakarta.servlet.AWSXRayServletFilter; import com.amazonaws.xray.strategy.jakarta.SegmentNamingStrategy; @Configuration public class WebConfig { @Bean public Filter TracingFilter() { return new AWSXRayServletFilter(SegmentNamingStrategy.dynamic("Scorekeep")); } }
对代码添加注释或实现接口
您的类必须使用 @XRayEnabled
注释添加注释,或实现 XRayTraced
接口。这将告知 AOP 系统包装受影响类的函数以进行 X-Ray 检测。
激活应用程序中的 X-Ray
要激活应用程序中的 X-Ray 跟踪,您的代码必须通过覆盖以下方法来扩展抽象类 BaseAbstractXRayInterceptor
。
-
generateMetadata
- 此函数允许对附加到当前函数跟踪的元数据进行自定义。默认情况下,执行函数的类名将记录在元数据中。如果您需要其他信息,则可添加更多数据。 -
xrayEnabledClasses
- 此函数为空,并且应保持此状态。它用作告知拦截程序要包装的方法的指示的主机。通过指定使用要跟踪的@XRayEnabled
添加注释的类来定义指示。以下指示语句告知拦截程序包装使用@XRayEnabled
注释添加注释的所有控制器 bean。@Pointcut(“@within(com.amazonaws.xray.spring.aop.XRayEnabled) && bean(*Controller)”)
如果项目使用的是 Spring Data JPA,请考虑从 AbstractXRayInterceptor
而非 BaseAbstractXRayInterceptor
进行扩展。
示例
以下代码扩展抽象类 BaseAbstractXRayInterceptor
。
@Aspect @Component public class XRayInspector extends BaseAbstractXRayInterceptor { @Override protected Map<String, Map<String, Object>> generateMetadata(ProceedingJoinPoint proceedingJoinPoint, Subsegment subsegment) throws Exception { return super.generateMetadata(proceedingJoinPoint, subsegment); } @Override @Pointcut("@within(com.amazonaws.xray.spring.aop.XRayEnabled) && bean(*Controller)") public void xrayEnabledClasses() {} }
以下代码是一个将由 X-Ray 检测的类。
@Service @XRayEnabled public class MyServiceImpl implements MyService { private final MyEntityRepository myEntityRepository; @Autowired public MyServiceImpl(MyEntityRepository myEntityRepository) { this.myEntityRepository = myEntityRepository; } @Transactional(readOnly = true) public List<MyEntity> getMyEntities(){ try(Stream<MyEntity> entityStream = this.myEntityRepository.streamAll()){ return entityStream.sorted().collect(Collectors.toList()); } } }
如果您已正确配置您的应用程序,则应看到应用程序的完整调用堆栈(从控制器向下至服务调用),如以下控制台屏幕截图所示。
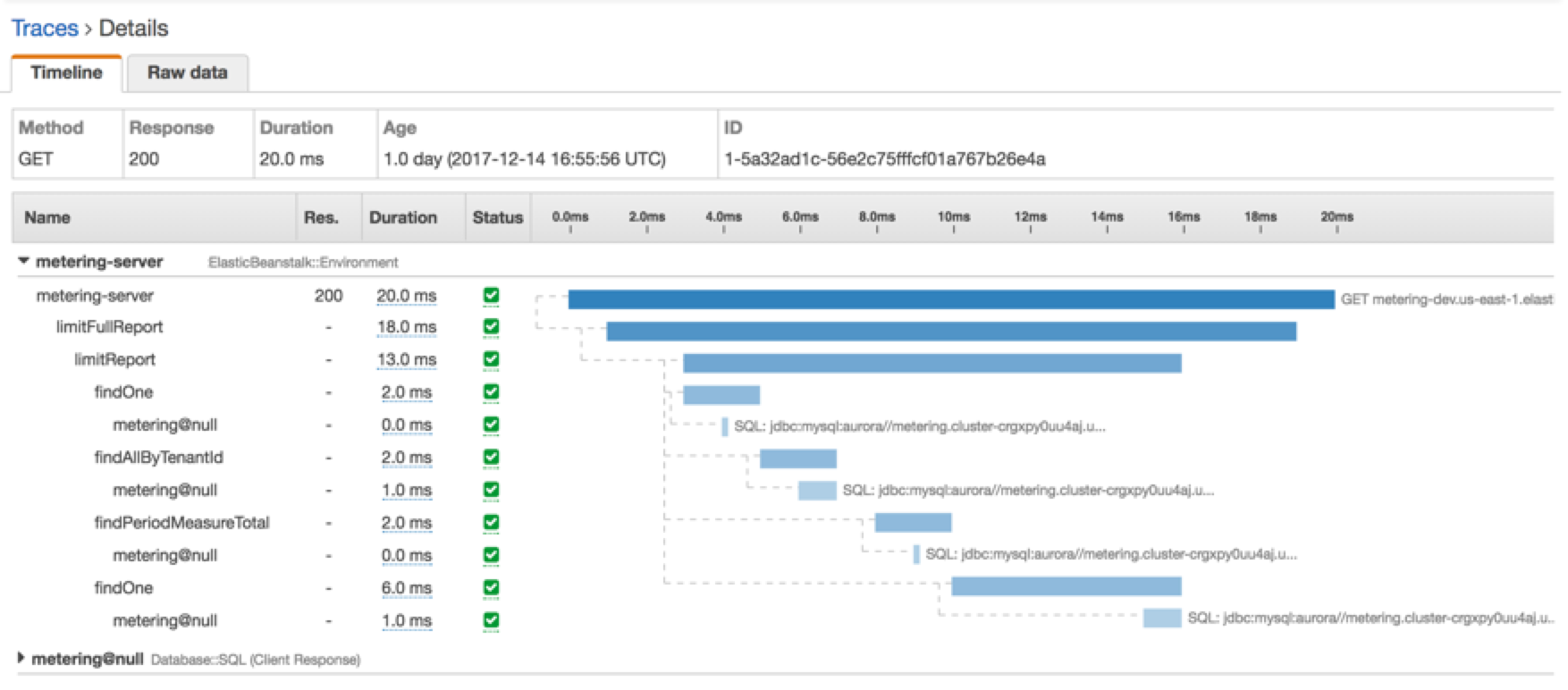