How authentication works with Amazon Cognito user pools and identity pools
When your customer signs in to an Amazon Cognito user pool, your application receives JSON web tokens (JWTs).
When your customer signs in to an identity pool, either with a user pool token or another provider, your application receives temporary Amazon credentials.
With user pool sign-in, you can implement authentication and authorization entirely with an Amazon SDK. If you don't want to build your own user interface (UI) components, you can invoke a prebuilt web UI (the hosted UI) or the sign-in page for your third-party identity provider (IdP).
This topic is an overview of some of the ways that your application can interact with Amazon Cognito to authenticate with ID tokens, authorize with access tokens, and access Amazon Web Services with identity pool credentials.
Topics
User pool API authentication and authorization with an Amazon SDK
Amazon has developed components for Amazon Cognito user pools, or Amazon Cognito identity provider, in a variety of developer frameworks. The methods built into these SDKs call the Amazon Cognito user pools API. The same user pools API namespace has operations for configuration of user pools and for user authentication. For a more thorough overview, see Using the Amazon Cognito user pools API and user pool endpoints.
API authentication fits the model where your applications have existing UI components and primarily rely on the user pool as a user directory. This design adds Amazon Cognito as a component within a larger application. It requires programmatic logic to handle complex chains of challenge and response.
This application doesn't need to implement a full OpenID Connect (OIDC) relying party implementation. Instead, it has the ability to decode and use JWTs. When you want access to the full set of user pool features for local users, build your authentication with the Amazon Cognito SDK in your development environment.
API authentication with custom OAuth scopes is less oriented toward external API authorization. To add custom scopes to an access token from API authentication, modify the token at runtime with a Pre token generation Lambda trigger.
The following diagram illustrates a typical sign-in session for API authentication.
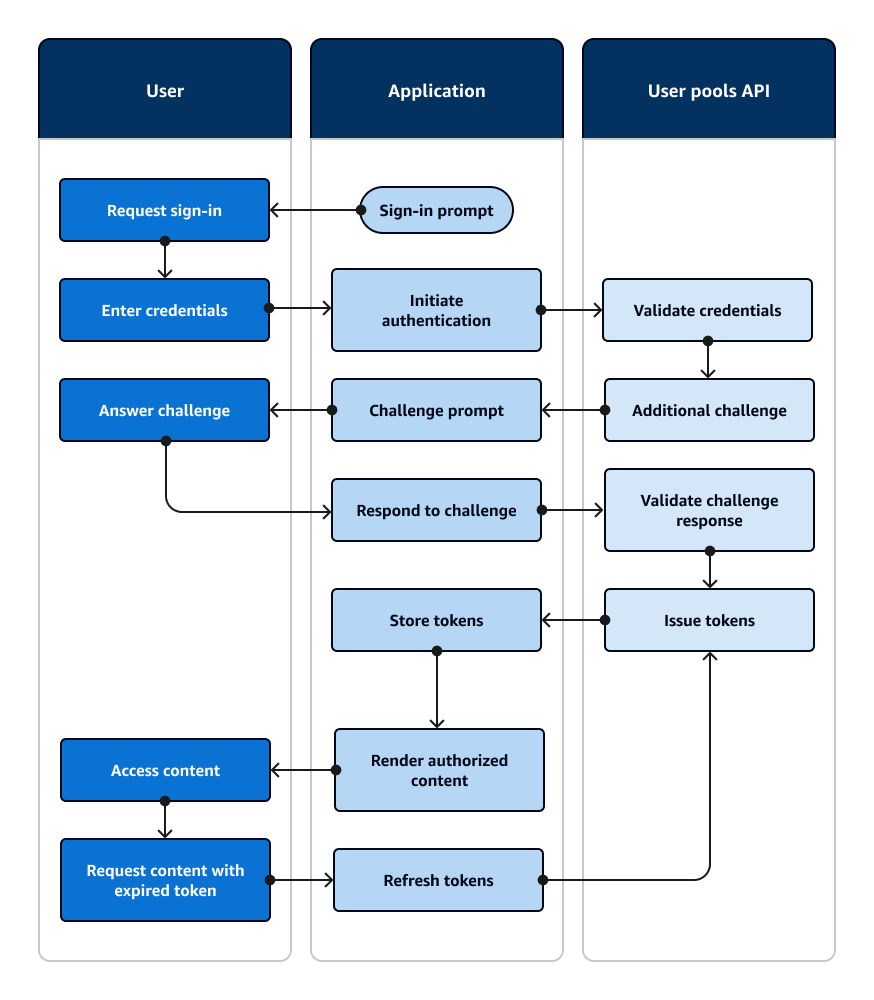
API authentication flow
-
A user accesses your application.
-
They select a "Sign in" link.
-
They enter their username and password.
-
The application invokes the method that makes an InitiateAuth API request. The request passes the user's credentials to a user pool.
-
The user pool validates the user's credentials and determines that the user has activated multi-factor authentication (MFA).
-
The user pool responds with a challenge that requests an MFA code.
-
The application generates a prompt that collects the MFA code from the user.
-
The application invokes the method that makes a RespondToAuthChallenge API request. The request passes the user's MFA code.
-
The user pool validates the user's MFA code.
-
The user pool responds with the user's JWTs.
-
The application decodes, validates, and stores or caches the user's JWTs.
-
The application displays the requested access-controlled component.
-
The user views their content.
-
Later, the user's access token has expired, and they request to view an access-controlled component.
-
The application determines that the user's session should persist. It invokes the InitiateAuth method again with the refresh token and retrieves new tokens.
Variants and customization
You can augment this flow with additional challenges—for example, your own custom authentication challenges. You can automatically restrict access for users whose passwords have been compromised, or whose unexpected sign-in characteristics might indicate a malicious sign-in attempt. This flow looks much the same for operations to sign up, update user attributes, and reset passwords. Most of these flows have duplicate public (client-side) and confidential (server-side) API operations.
Related resources
User pool authentication with the hosted UI
The hosted UI is a website that is linked to your user pool and app client. It can perform sign-in, sign-up, and password-reset operations for your users. An application with a hosted UI component for authentication can require less developer effort to implement. An application can skip UI components for authentication and invoke the hosted UI in the user's browser.
Applications collect users' JWTs with a web or app redirect location. Applications that implement the hosted UI can connect to user pools for authentication as if they were an OpenID Connect (OIDC) IdP.
Hosted UI authentication fits the model where applications need an authorization server, but don't need features like custom authentication, identity pools integration, or user attribute self-service. When you want to use some of these advanced options, you can implement them with a user pools component for an SDK.
Hosted UI and third-party IdP authentication models, with a primary reliance on OIDC implementation, are best for advanced authorization models with OAuth 2.0 scopes.
The following diagram illustrates a typical sign-in session for hosted UI authentication.
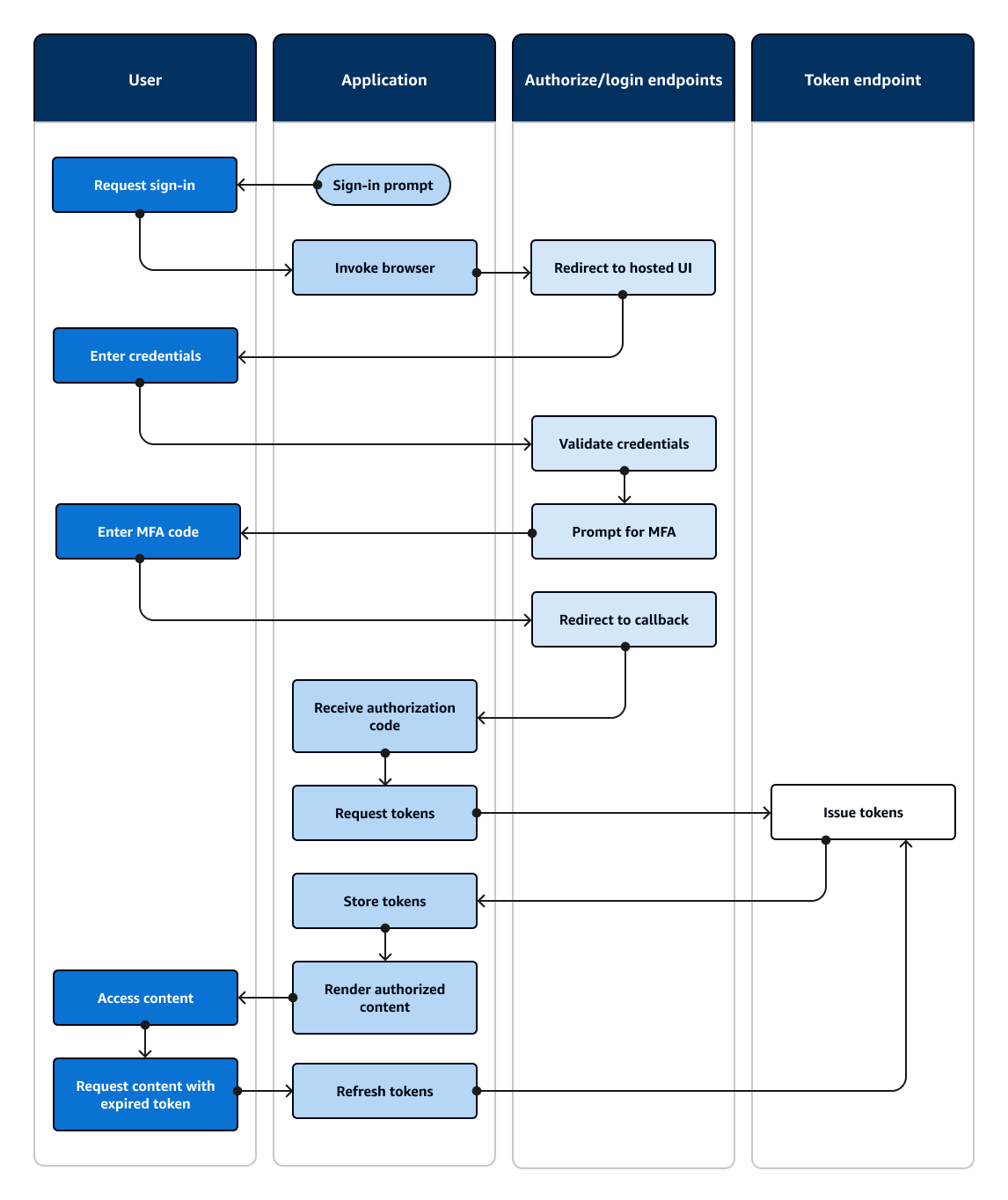
Hosted UI authentication flow
-
A user accesses your application.
-
They select a "Sign in" link.
-
The application directs the user to a hosted UI sign-in prompt.
-
They enter their username and password.
-
The user pool validates the user's credentials and determines that the user has activated multi-factor authentication (MFA).
-
The hosted UI prompts the user to enter an MFA code.
-
The user enters their MFA code.
-
The hosted UI redirects the user to the application.
-
The application collects the authorization code from the URL request parameter that the hosted UI appended to the callback URL.
-
The application requests tokens with the authorization code.
-
The token endpoint returns JWTs to the application.
-
The application decodes, validates, and stores or caches the user's JWTs.
-
The application displays the requested access-controlled component.
-
The user views their content.
-
Later, the user's access token has expired, and they request to view an access-controlled component.
-
The application determines that the user's session should persist. It requests new tokens from the token endpoint with the refresh token.
Variants and customization
You can customize the look and feel of the hosted UI with CSS in any app client. You can also configure app clients with their own identity providers, scopes, access to user attributes, and advanced security configuration.
Related resources
User pool authentication with a third-party identity provider
Sign-in with an external identity provider (IdP), or federated authentication, is a similar model to the hosted UI. Your application is an OIDC relying party to your user pool, while your user pool serves as a passthrough to an IdP. The IdP can be a consumer user directory like Facebook or Google, or it can be a SAML 2.0 or OIDC enterprise directory like Azure.
Instead of the hosted UI in the user's browser, your application invokes a redirect endpoint on the user pool authorization server. From the user's view, they choose the sign-in button in your application. Then their IdP prompts them to sign in. Like with hosted UI authentication, an application collects JWTs at a redirect location in the app.
Authentication with a third-party IdP fits a model where users might not want to come up with a new password when they sign up for your application. Third-party authentication can be added with low effort to an application that's implemented hosted UI authentication. In effect, the hosted UI and third-party IdPs produce a consistent authentication outcome from minor variations in what you invoke in users' browsers.
Like hosted UI authentication, federated authentication is best for advanced authorization models with OAuth 2.0 scopes.
The following diagram illustrates a typical sign-in session for federated authentication.
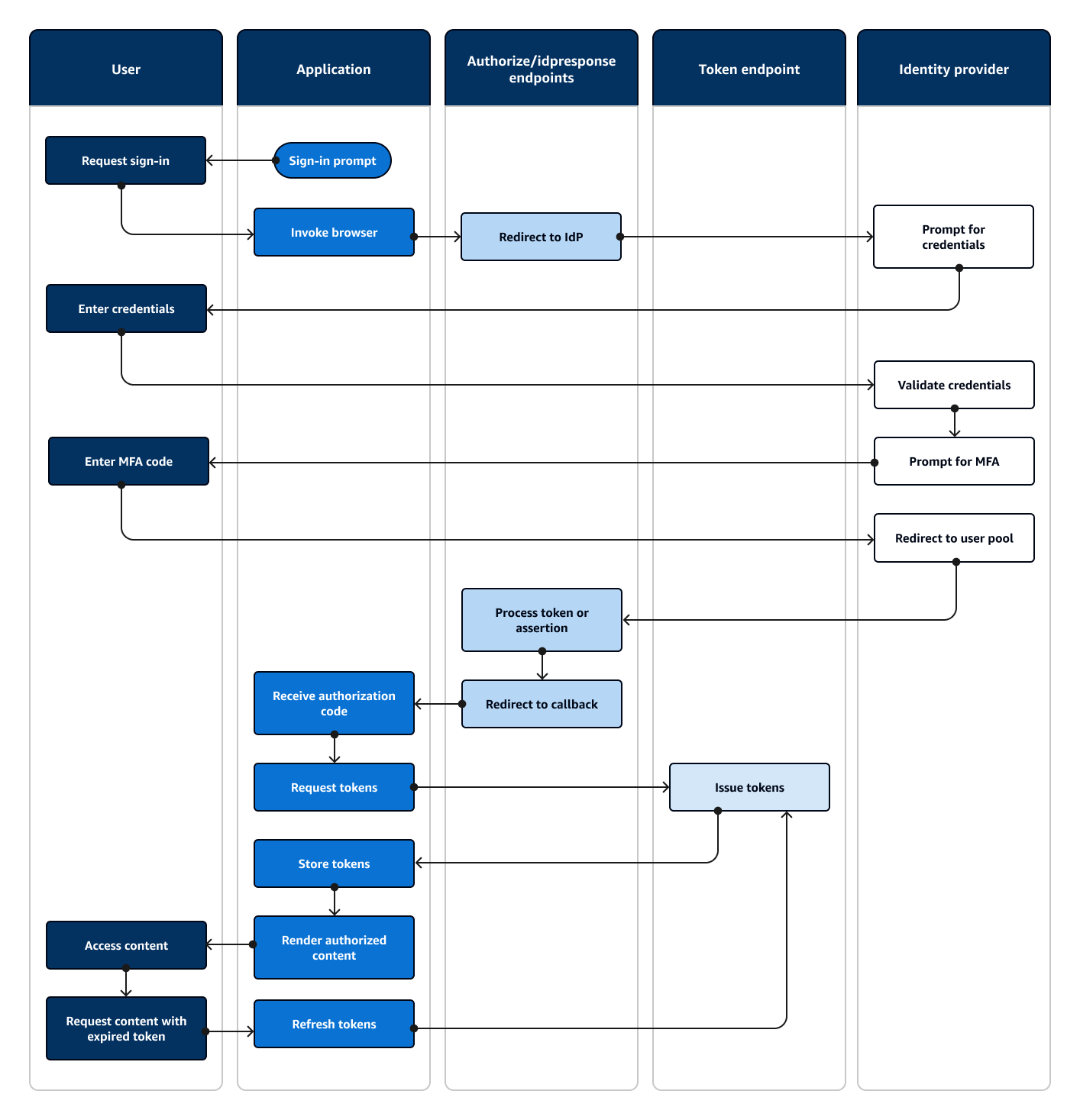
Federated authentication flow
-
A user accesses your application.
-
They select a "Sign in" link.
-
The application directs the user to a sign-in prompt with their IdP.
-
They enter their username and password.
-
The IdP validates the user's credentials and determines that the user has activated multi-factor authentication (MFA).
-
The IdP prompts the user to enter an MFA code.
-
The user enters their MFA code.
-
The IdP redirects the user to the user pool with a SAML response or an authorization code.
-
If the user passed an authorization code, the user pool silently exchanges the code for IdP tokens. The user pool validates the IdP tokens and redirects the user to the application with a new authorization code.
-
The application collects the authorization code from the URL request parameter that the user pool appended to the callback URL.
-
The application requests tokens with the authorization code.
-
The token endpoint returns JWTs to the application.
-
The application decodes, validates, and stores or caches the user's JWTs.
-
The application displays the requested access-controlled component.
-
The user views their content.
-
Later, the user's access token has expired, and they request to view an access-controlled component.
-
The application determines that the user's session should persist. It requests new tokens from the token endpoint with the refresh token.
Variants and customization
You can initiate federated authentication in the hosted UI, where users can choose from a list of IdPs that you assigned to your app client. The hosted UI can also prompt for an email address and automatically route a user's request to the corresponding SAML IdP. Authentication with a third-party identity provider doesn't require user interaction with the hosted UI. Your application can add a request parameter to a user's authorization server request and cause the user to silently redirect to their IdP sign-in page.
Related resources
Identity pool authentication
An identity pool is a component for your application that is distinct from a user pool in function, API namespace, and SDK model. Where user pools offer token-based authentication and authorization, identity pools offer authorization for Amazon Identity and Access Management (IAM).
You can assign a set of IdPs to identity pools and sign in users with them. User pools are closely integrated as identity pool IdPs and give identity pools the most options for access control. At the same time, there is a wide selection of authentication options for identity pools. User pools join SAML, OIDC, social, developer, and guest identity sources as routes to temporary Amazon credentials from identity pools.
Authentication with an identity pool is external—it follows one of the previously illustrated user pool flows, or a flow that you develop independently with another IdP. After your application performs initial authentication, it passes the proof to an identity pool and receives a temporary session in return.
Authentication with an identity pool fits a model where you enforce the access control for application assets and data in Amazon Web Services with IAM authorization. Like with API authentication in user pools, a successful application includes Amazon SDKs for each of the services that you want to access for your users' benefit. Amazon SDKs apply the credentials from identity pool authentication as signatures to API requests.
The following diagram illustrates a typical sign-in session for identity pool authentication with an IdP.
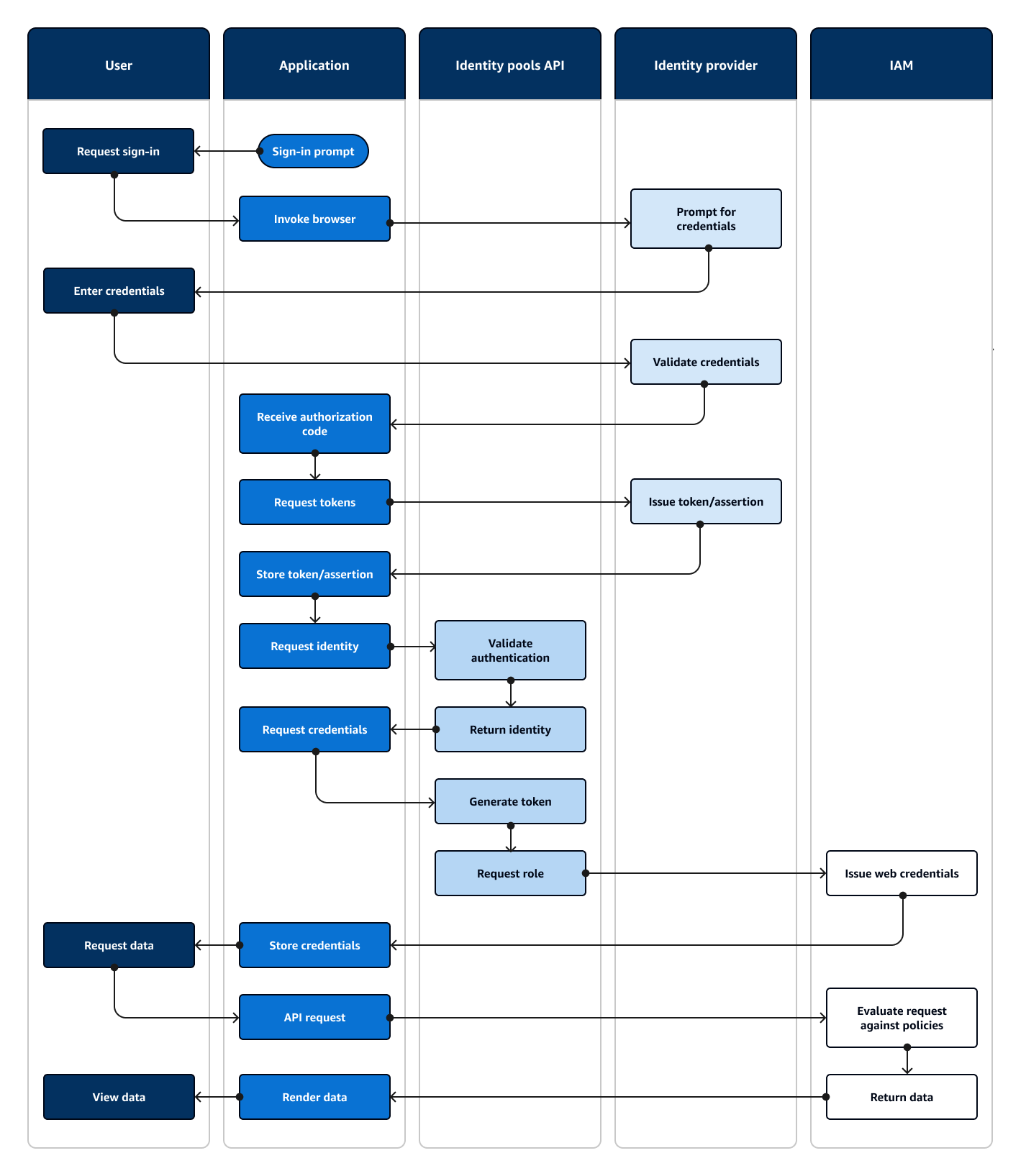
Federated authentication flow
-
A user accesses your application.
-
They select a "Sign in" link.
-
The application directs the user to a sign-in prompt with their IdP.
-
They enter their username and password.
-
The IdP validates the user's credentials.
-
The IdP redirects the user to the application with a SAML response or an authorization code.
-
If the user passed an authorization code, the application exchanges the code for IdP tokens.
-
The application decodes, validates, and stores or caches the user's JWTs or assertion.
-
The application invokes the method that makes a GetId API request. It passes the user's token or assertion and requests an identity ID.
-
The identity pool validates the token or assertion against configured identity providers.
-
The identity pool returns an identity ID.
-
The application invokes the method that makes a GetCredentialsForIdentity API request. It passes the user's token or assertions and requests an IAM role.
-
The identity pool generates a new JWT. The new JWT contains claims that request an IAM role. The identity pool determines the role based on the user's request and the role-selection criteria in the identity pool configuration for the IdP.
-
Amazon Security Token Service (Amazon STS) responds to the AssumeRoleWithWebIdentity request from the identity pool. The response contains API credentials for a temporary session with an IAM role.
-
The application stores the session credentials.
-
The user takes an action in the app that requires access-protected resources in Amazon.
-
The application applies the temporary credentials as signatures to API requests for the required Amazon Web Services.
-
IAM evaluates the policies attached to the role in the credentials. It compares them to the request.
-
The Amazon Web Service returns the requested data.
-
The application renders the data in the user's interface.
-
The user views the data.
Variants and customization
To visualize authentication with a user pool, insert one of the previous user-pool overviews after the Issue token/assertion step. Developer authentication replaces all steps before Request identity with a request signed by developer credentials. Guest authentication also skips straight to Request identity, doesn't validate authentication, and returns credentials for a limited-access IAM role.