Pre authentication Lambda trigger
Amazon Cognito invokes this trigger when a user attempts to sign in so that you can create custom validation that performs preparatory actions. For example, you can deny the authentication request or record session data to an external system.
Note
This Lambda trigger doesn't activate when a user doesn't exist unless the
PreventUserExistenceErrors
setting of a user pool app client is set to
ENABLED
. Renewal of an existing authentication session also doesn't
activate this trigger.
Flow overview
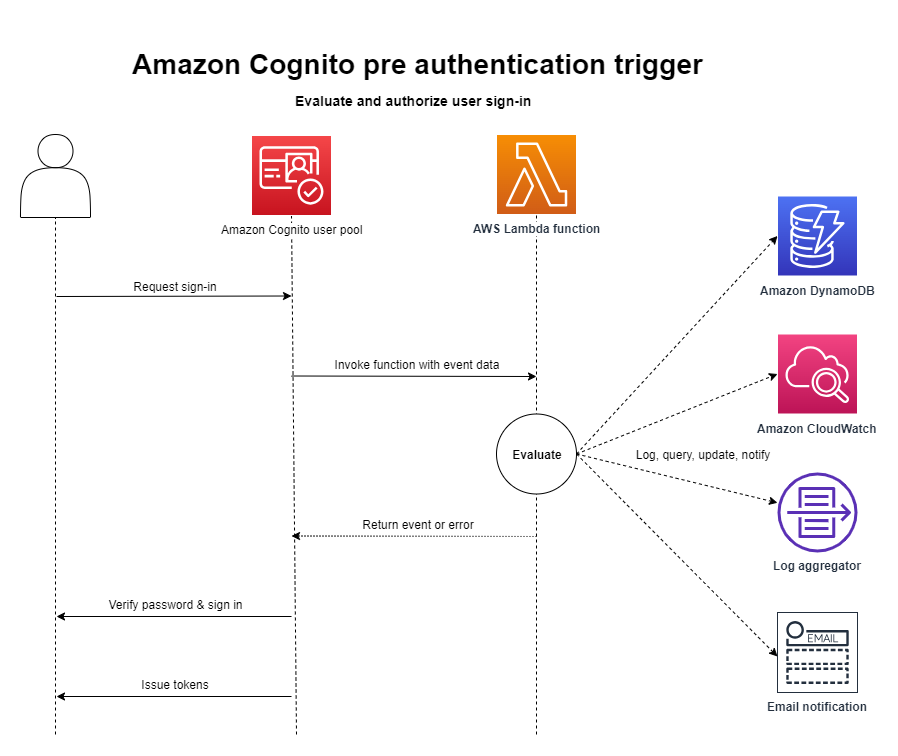
The request includes client validation data from the ClientMetadata
values that your app passes to the user pool InitiateAuth
and
AdminInitiateAuth
API operations.
For more information, see An example authentication session.
Pre authentication Lambda trigger parameters
The request that Amazon Cognito passes to this Lambda function is a combination of the parameters below and the common parameters that Amazon Cognito adds to all requests.
Pre authentication request parameters
- userAttributes
-
One or more name-value pairs that represent user attributes.
- userNotFound
-
When you set
PreventUserExistenceErrors
toENABLED
for your user pool client, Amazon Cognito populates this Boolean. - validationData
-
One or more key-value pairs that contain the validation data in the user's sign-in request. To pass this data to your Lambda function, use the ClientMetadata parameter in the InitiateAuth and AdminInitiateAuth API actions.
Pre authentication response parameters
Amazon Cognito doesn't process any added information that your function returns in the response. Your function can return an error to reject the sign-in attempt, or use API operations to query and modify your resources.
Pre authentication example
This example function prevents users from signing in to your user pool with a specific app client. Because the pre authentication Lambda function doesn't invoke when your user has an existing session, this function only prevents new sessions with the app client ID that you want to block.
Amazon Cognito passes event information to your Lambda function. The function then returns the same event object to Amazon Cognito, with any changes in the response. In the Lambda console, you can set up a test event with data that is relevant to your Lambda trigger. The following is a test event for this code sample: