HTTP clients
You can change the HTTP client to use for your service client as well as change the default configuration for HTTP clients with the Amazon SDK for Java 2.x. This section discusses HTTP clients and settings for the SDK.
HTTP clients available in the SDK for Java
Synchronous clients
Synchronous HTTP clients in the SDK for Java implement the SdkHttpClientS3Client
or the DynamoDbClient
, requires the use of a
synchronous HTTP client. The Amazon SDK for Java offers three synchronous HTTP clients.
- ApacheHttpClient (default)
-
ApacheHttpClient
is the default HTTP client for synchronous service clients. For information about configuring the ApacheHttpClient
, see Configure the Apache-based HTTP client. - AwsCrtHttpClient
-
AwsCrtHttpClient
provides high throughput and non-blocking IO. It is built on the Amazon Common Runtime (CRT) Http Client. For information about configuring the AwsCrtHttpClient
and using it with service clients, see Configure Amazon CRT-based HTTP clients. - UrlConnectionHttpClient
-
To minimize the number of jars and third-party libraries you application uses, you can use the UrlConnectionHttpClient
. For information about configuring the UrlConnectionHttpClient
, see Configure the URLConnection-based HTTP client.
Asynchronous clients
Asynchronous HTTP clients in the SDK for Java implement the SdkAsyncHttpClientS3AsyncClient
or the DynamoDbAsyncClient
, requires the use
of an asynchronous HTTP client. The Amazon SDK for Java offers two asynchronous HTTP
clients.
- NettyNioAsyncHttpClient (default)
-
NettyNioAsyncHttpClient
is the default HTTP client used by asynchronous clients. For information about configuring the NettyNioAsyncHttpClient
, see Configure the Netty-based HTTP client. - AwsCrtAsyncHttpClient
-
The AwsCrtAsyncHttpClient
is based on the Amazon Common Runtime (CRT) HTTP Client. For information about configuring the AwsCrtAsyncHttpClient
, see Configure Amazon CRT-based HTTP clients.
HTTP client recommendations
Several factors come into play when you choose an HTTP client implementation. Use the following information to help you decide.
Recommendation flowchart
The following flowchart provides general guidance to help you determine which HTTP client to use.
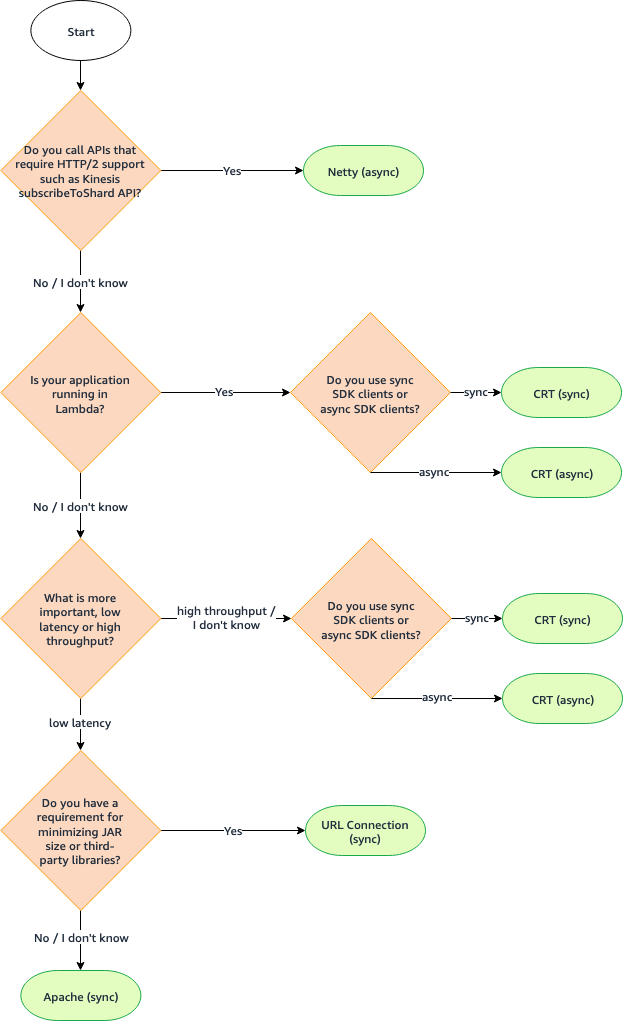
HTTP client comparison
The following table provides detailed information for each HTTP client.
HTTP client | Sync or async | When to use | Limitation/drawback |
---|---|---|---|
Apache-based HTTP client
(default sync HTTP client) |
Sync | Use it if you prefer low latency over high throughput | Slower startup time compared to other HTTP clients |
URLConnection-based HTTP client | Sync | Use it if you have a hard requirement for limiting third-party dependencies | Does not support the HTTP PATCH method, required by some APIS like Amazon APIGateway Update operations |
Amazon CRT-based sync HTTP client1 | Sync |
• Use it if your application is running in Amazon Lambda • Use it if you prefer high throughput over low latency • Use it if you prefer sync SDK clients |
The following Java system properties are not supported:
|
Netty-based HTTP client
(default async HTTP client) |
Async |
• Use it if your application invokes APIs that require HTTP/2
support such as Kinesis API SubscribeToShard |
Slower startup time compared to other HTTP clients |
Amazon CRT-based async HTTP client1 | Async | • Use it if your application is running in Amazon Lambda • Use it if you prefer high throughput over low latency • Use it if you prefer async SDK clients |
• Does not support service clients that require HTTP/2 support
such as The following Java system properties are not supported:
|
1Because of their added benefits, we recommend that you use the Amazon CRT-based HTTP clients if possible.
Smart configuration defaults
The Amazon SDK for Java 2.x (version 2.17.102 or later) offers a smart configuration defaults feature. This feature optimizes two HTTP client properties along with other properties that don't affect the HTTP client.
The smart configuration defaults set sensible values for the
connectTimeoutInMillis
and tlsNegotiationTimeoutInMillis
properties based on a defaults mode value that you provide. You choose the defaults mode
value based on your application's characteristics.
For more information about smart configuration defaults and how to choose the defaults mode value that is best suited for your applications, see the Amazon SDKs and Tools Reference Guide.
Following are four ways to set the defaults mode for your application.
If you set the defaults mode globally with the system property, environment variable, or Amazon config file, you can override the settings when you build an HTTP client.
When you build an HTTP client with the httpClientBuilder()
method, settings
apply only to the instance that you are building. An example of this is shown here. The Netty-based HTTP client in this
example overrides any default mode values set globally for
connectTimeoutInMillis
and
tlsNegotiationTimeoutInMillis
.